“I’m parsing data from an email but the information is always on the next line after specific string, how to extract it in Power Automate?”
When you parse an email, there’re at least two ways how the data can be formatted – a block of text or a table. While it’s quite easy to find the desired information in a text, it’s a bit more complicated with the table. Converting an HTML table into text will turn each column into a separate line. The identifier is on one line, the value on the other…
In the past I used xpath(…), but that solution feels too complicated now. This article will show you another, much simpler approach. A few steps to find a specific line in an array, and extract the value from the next one.
Start from the array
The beginning is the as with a block of text – you need an array. If you already have an array, you can skip this step. If you’re parsing an email, turn it into a text and split by the new line character.
split(body('Html_to_text'),decodeUriComponent('%0A%0A'))
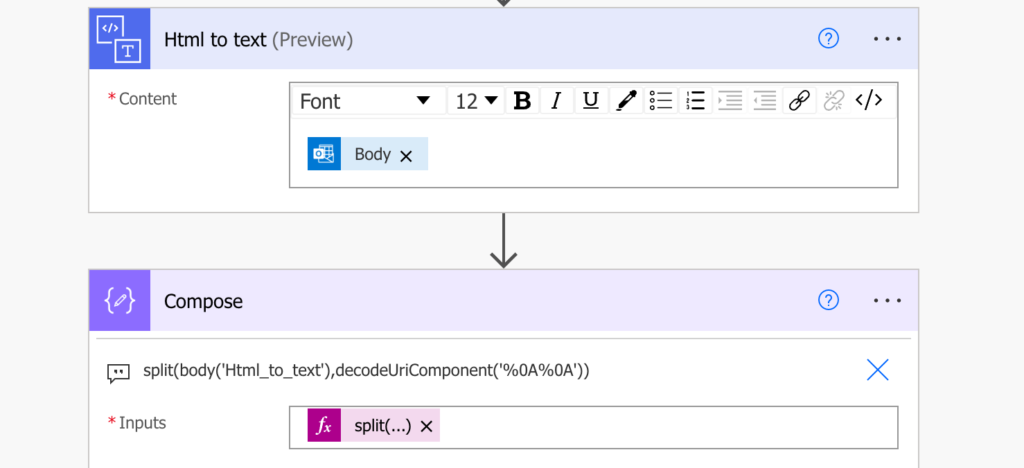
You’ll get an array where each line represents a table cell. In the example below there’s the identifier (Name, Company, Email) with the data on the next line.
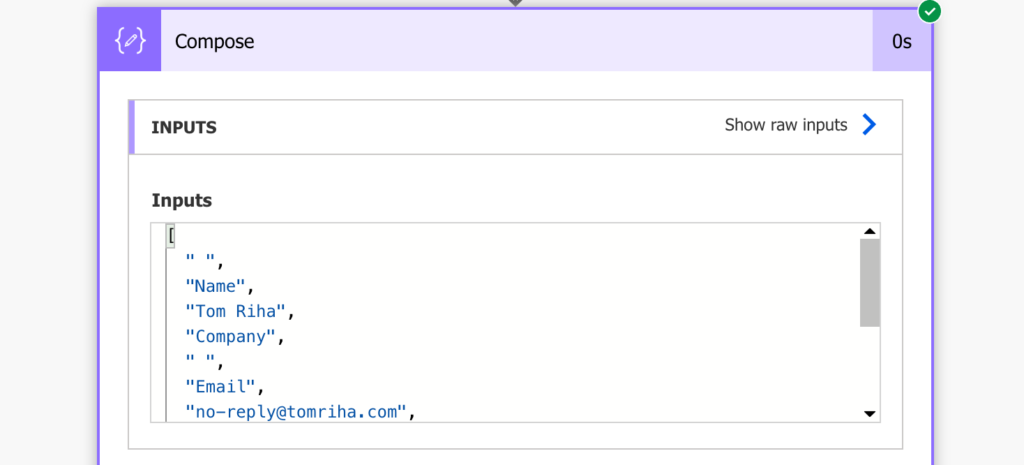
Extract data from “the next line”
If everything was on the same line, you’d extract it easily with the ‘Filter array’ action. But it’s not. Filtering the array won’t help.
Instead, turn it into a single string with a separator, e.g. ##, using the join(…) expression.
join(outputs('Compose'),'##')
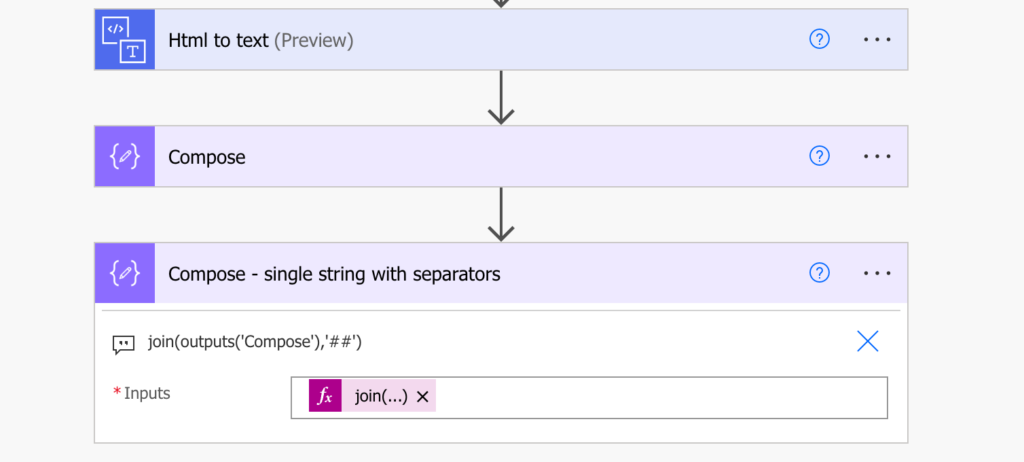
It’s this long string with a clear distinction between the data rows where you can do the extraction.
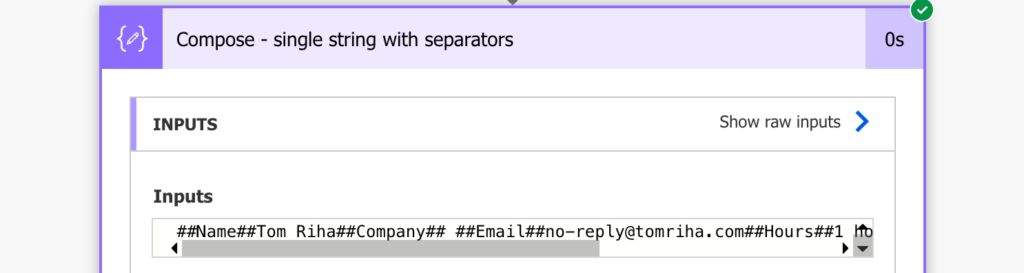
Firstly, split it by the data identifier and the separator, e.g. the Name##. That way you can remove everything before that, including the identifier.
split(outputs('Compose_-_single_string_with_separators'),'Name##')[1]
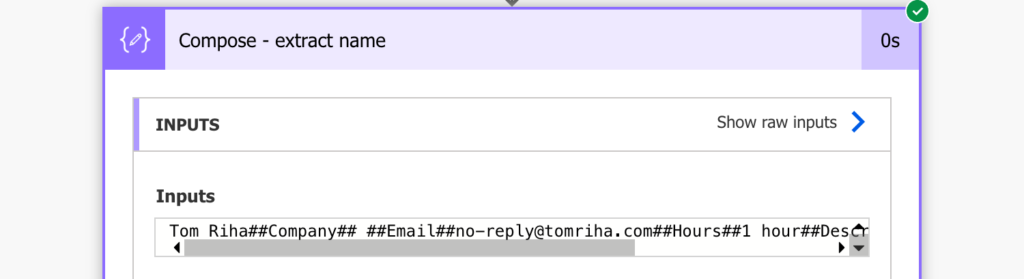
Now, when the string starts with the actual value, you have to remove everything after. Since you added a separator between the rows, you can now use it to take everything before its first occurrence.
Split(…) it again, this time using only the separator and keeping the first part.
split(split(outputs('Compose_-_single_string_with_separators'),'Name##')[1], '##')[0]
That’s it, you just extracted the value on the next line after ‘Name’.
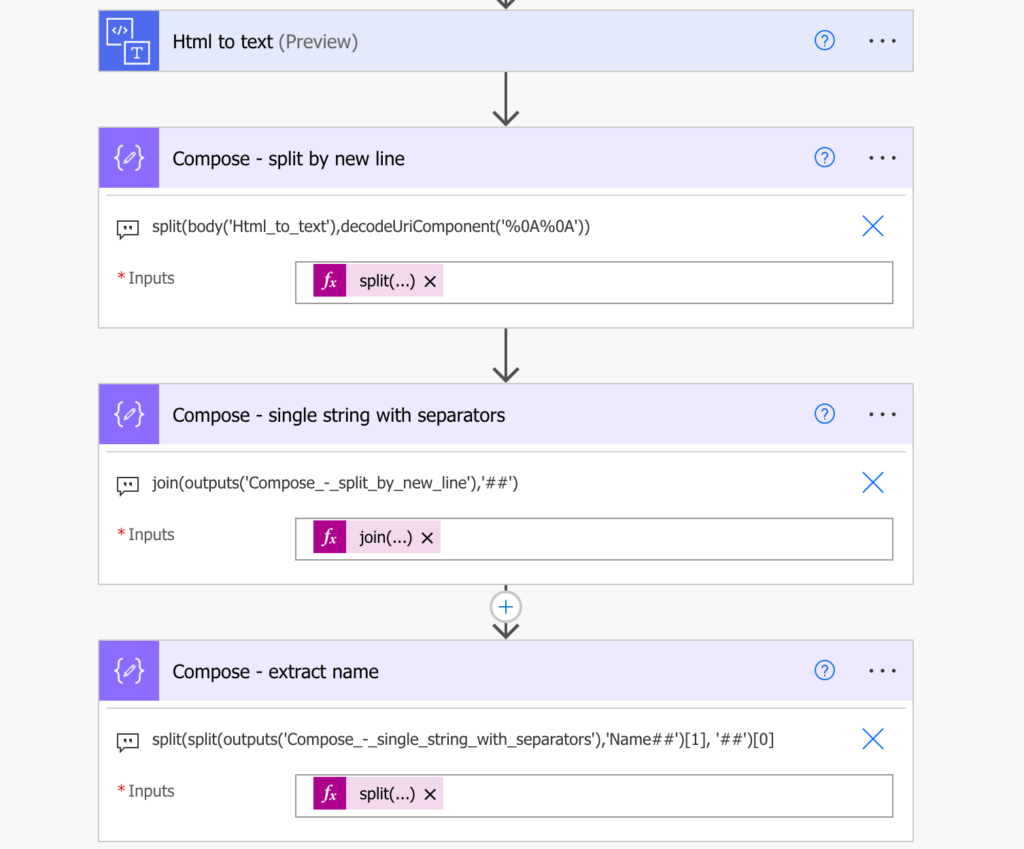
Summary
There’re two options to extract a specific piece of data in a flow – the ‘Filter array’ action or an expression. The ‘Filter array’ is great if everything is on a single line, but if it’s more complicated, if you need to get the next line after an identifier, it’s better to combine a few Power Automate expressions. Instead of an array that’s hard to dynamically navigate turn it into a string. Then it’s just a few split(…) expressions with indexes that’ll give you the value.