“I need to send an email to thousands of users but Exchange allows me to use only 500 recipients for an email, how can I split them into batches of 500 with Power Automate?”
If you’re sending emails to individual recipients, you might encounter the Exchange limit. There’s a maximum number of recipients for each email that’s set to 500 by default. If you want to send the same email to more recipients, you’ll have to either increase the limit (up to 1000), or split it into multiple emails. This post describes the second option – how to split the recipients into smaller batches of 500.
Create an array with the emails
The first step is to convert the email addresses into an array. If it’s a list of semicolon separated emails, split(…) it by the semicolon character, e.g.
split(<list of emails>, ';')
If it’s an array of objects, e.g. SharePoint items, then ‘Select’ only the email addresses.
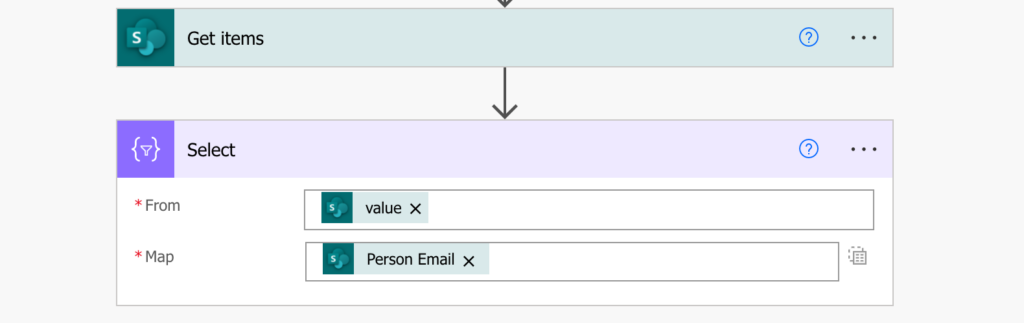
Updated Oct 2022: Use the chunk(…) expression
Power Automate has a new expression chunk(…) that’ll split an array into pieces. It has two parameters:
chunk(<array>, <chunkSize>)
Since you can handle 500 emails at once, the chunkSize will be 500:
chunk(body('Select'),500)
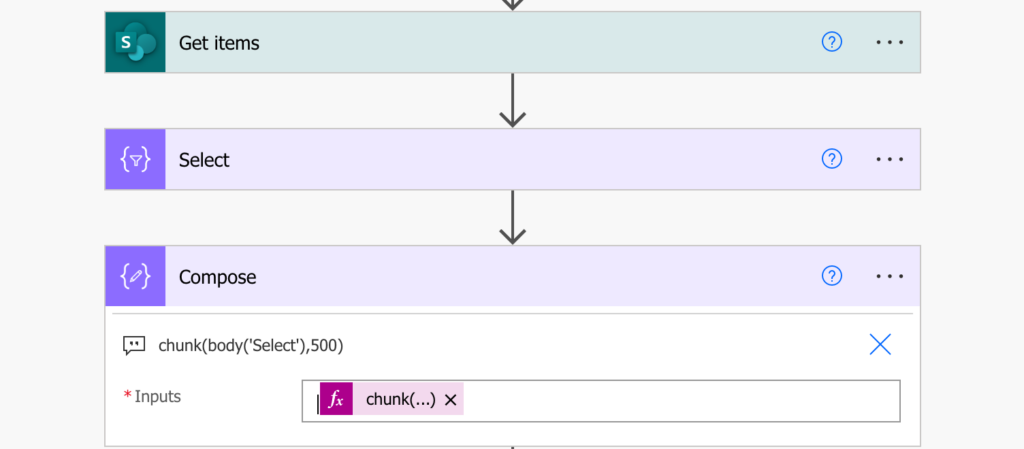
The expression will split the array into multiple arrays of arrays – loop through the chunks, join(…) the emails addresses in each of them and send the email.
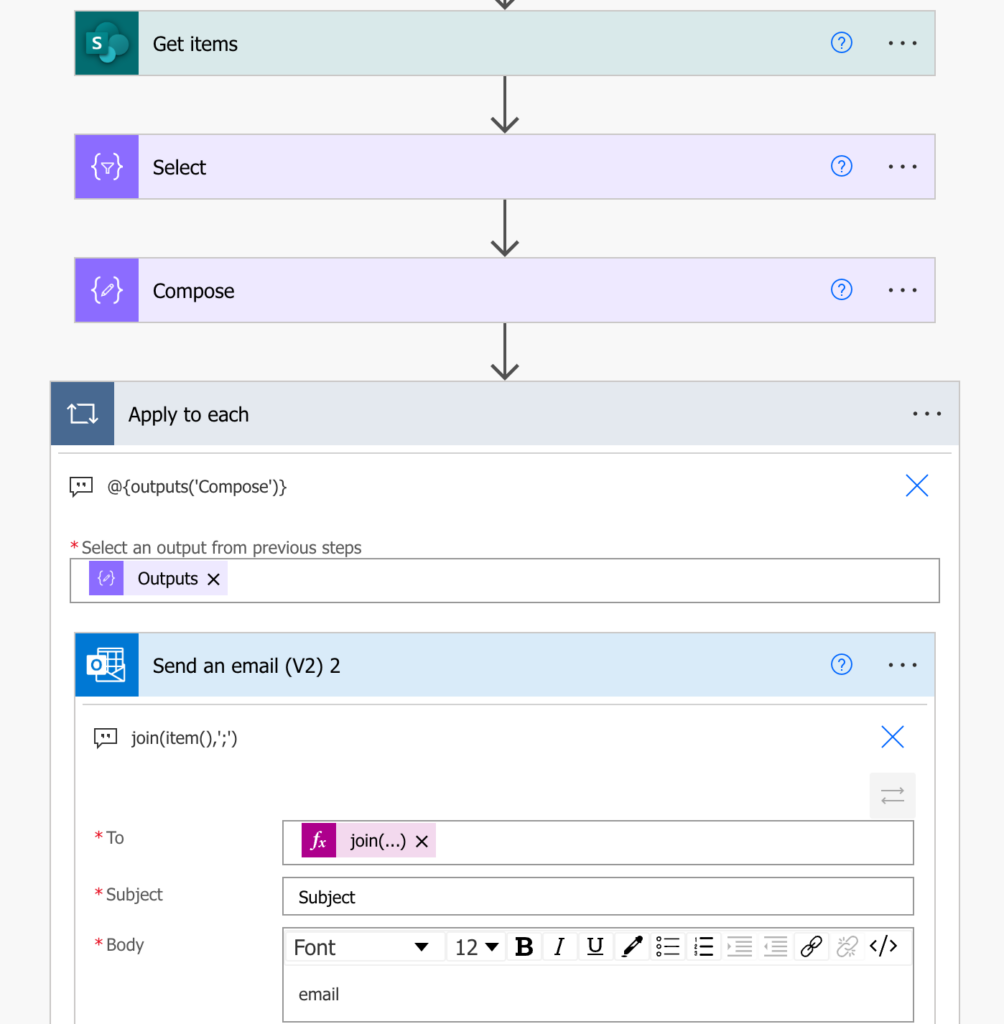
And that’s it. Much easier than the original approach which you can still see below using the skip(…) and take(…) concept.
The old approach: Take a piece of an array
For the actual split into batches you’ll need the skip(…) and take(…) expressions.
Take(…) expression
The take(…) expression will take the specified amount of items from an array. The array is the first parameter, number of items to take is the second one. For example, to take 500 items (emails) from an array:
take(<array with emails>, 500)
Note: <…> is a placeholder, replace it including the < and >.
But this will always take the first 500 emails. To process all the emails you must always skip those that were already processed. That’s what the skip(…) expression will do.
Skip(…) expression
The skip(…) expression will skip items in an array. You enter the array as the first parameter and the number of items to skip as the second one, e.g.
skip(<array with emails>, 10)
Note: <…> is a placeholder, replace it including the < and >
The expression above will skip the first 10 emails and return the rest. But here you don’t have a fixed number of emails to skip. At first you want to skip 0 and process the first 500, then skip that 500 and take another 500, followed by skipping 1000 and taking another 500. That’s why you’ll need a number variable – let’s call it var_processed and set it to 0.
This variable will serve as a counter of the processed emails.
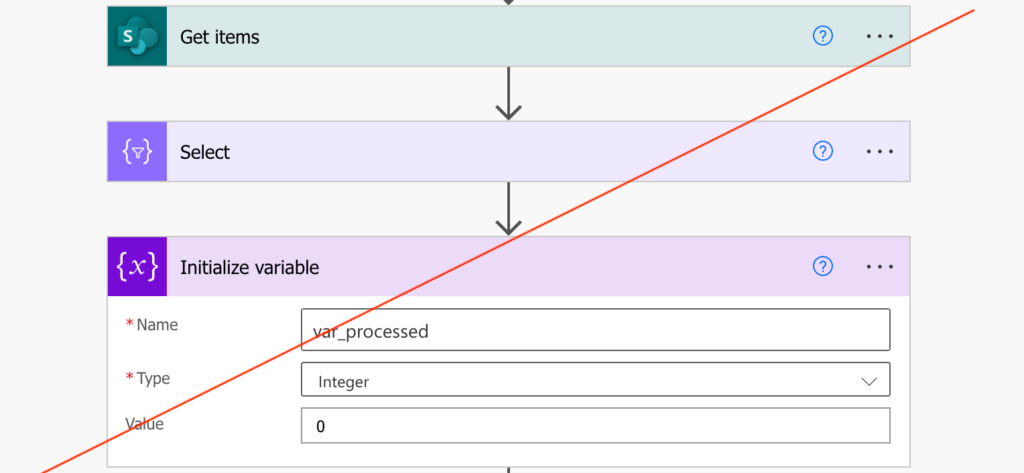
Process the batches of 500 emails
As already mentioned, you don’t want to take always the same 500 emails. You want to move in the array.
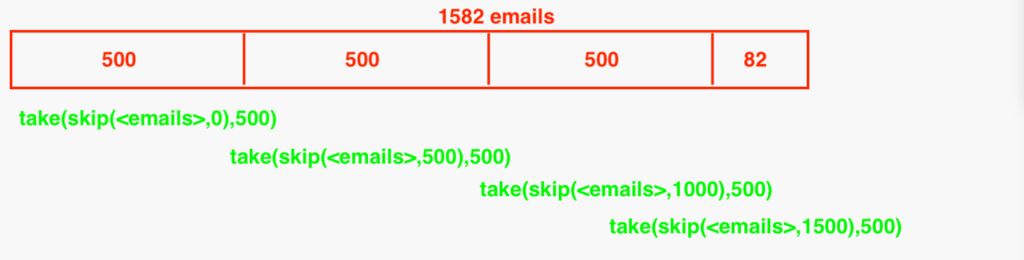
That can be done using the same expression while changing the number of emails to skip. That means a loop with the ‘Do until’ action. Keep moving in the array until the number of processed items (var_processed) is greater or equal to the count of all emails (length(…)).
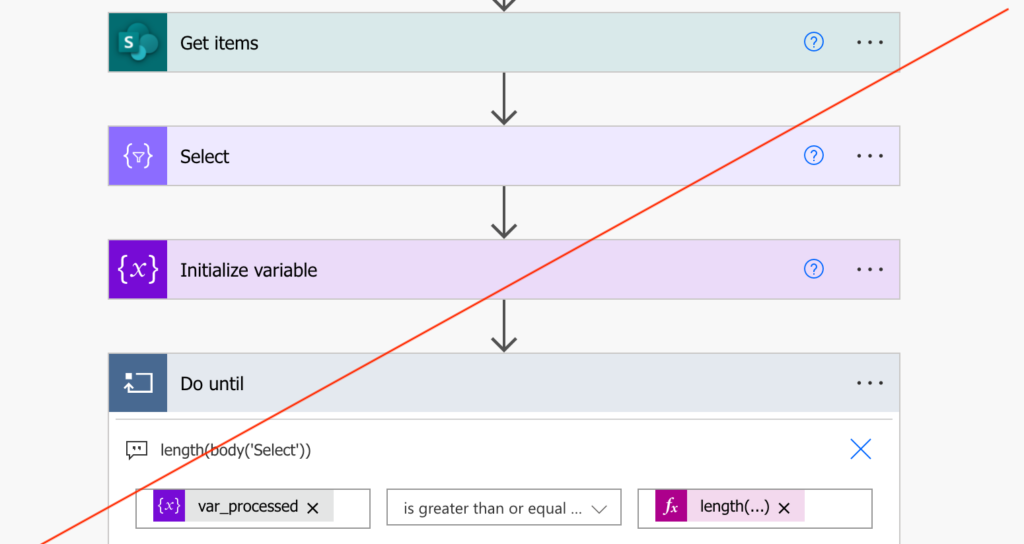
In each loop take the next 500 emails…
take(skip(body('Select'),variables('var_processed')),500)
…join them with the semicolon, and use them to send the email.
join(take(skip(body('Select'),variables('var_processed')),500),';')
Then increment the variable to skip the already processed 500 emails.
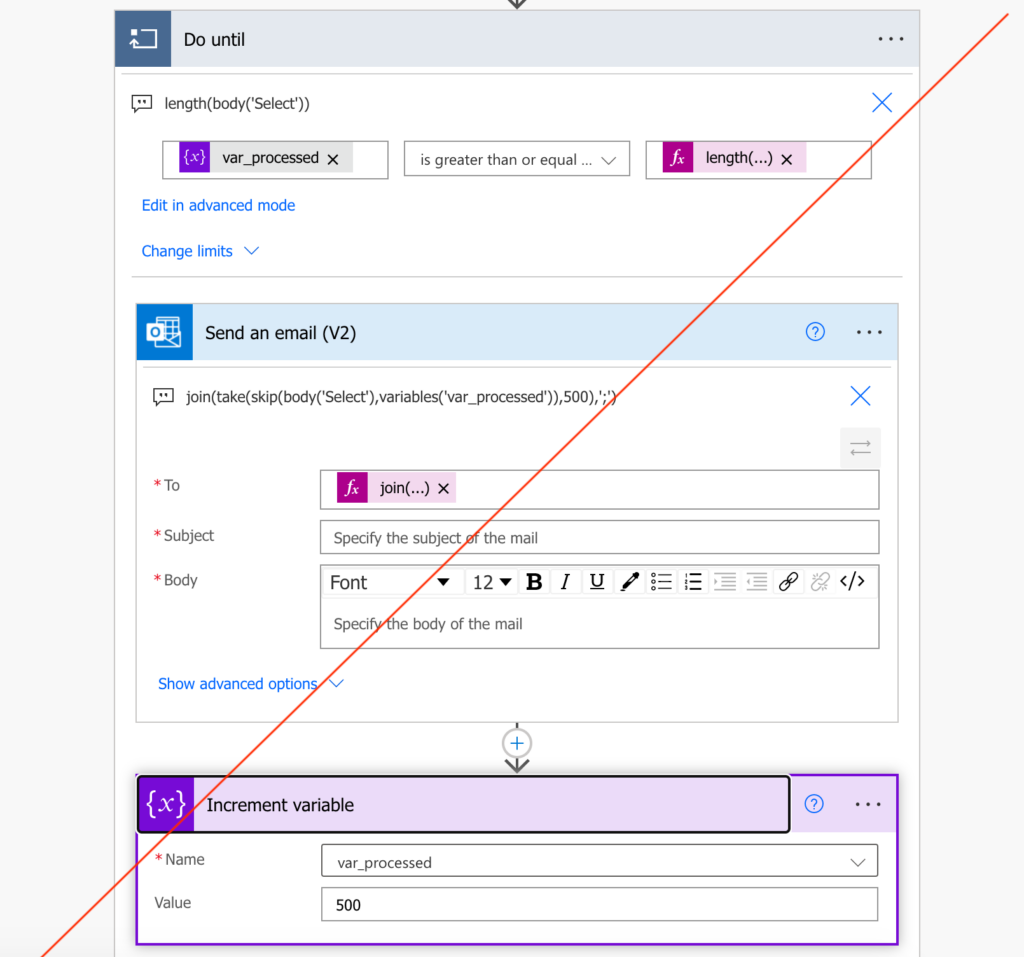
Summary
If you’re sending an email via Power Automate to more than 500 recipients, you’ve got two options. You can either increase the limit (if you’ve got administrator permissions and if 1000 is enough) or you’ll have to split it into multiple emails. Using a loop, variable, and the take(…) and skip(…) expressions you can split the recipients into batches, and process them within the default limitations.
Or you can use the new expression chunk(…) and split it in a single step.
I have two questions:
1) What did you write for the join expression.
2) I am experience errors at the join stage.
Error Discription:
The ‘inputs.parameters’ of workflow operation ‘Send_an_email_from_a_shared_mailbox_(V2)’ of type ‘OpenApiConnection’ is not valid. Error details: Input parameter ’emailMessage/Bcc’ is required to be of type ‘String/email’. The runtime value ‘”{\”Email\”:\”2367002@***.com\”};
Hello Tobi,
check the ‘Select’ action configuration, in your case it seems to return an object, but you want to return only the single value ‘… Email’.
hi
I have the same issue and i used your chunk and join exporession . It works fine as long as the body and subject is static but my issue is i have to send these emails multiple times. as i have various email templates and based on what email template is called in the flow, it needs to take the body and subject from that template . But its not working . How would i resolve that issue using chunk and join
Hello sandhya,
just repeat the actions for each body/subject.
If i use chunk operation i am targetting furst 500 emails what about if i have 600 how to target the rest 100 email via chunk piece
Hello Jacqui,
chunk(…) will return an array where you can navigate as in any other array: https://tomriha.com/how-to-navigate-in-an-array-within-power-automate-flow/